Summary of “Grids: positioning grid items”
In order to create a block using a grid container, you need to assign it the corresponding value for the display
property:
.container {
display: grid;
}
The grid items are arranged along a two-dimensional grid. In other words, the grid consists of rows and columns that are located between the lines, which are numbered in sequence.
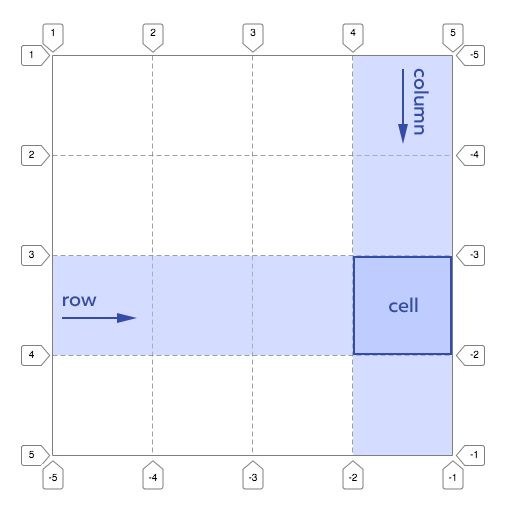
To place an element on the grid, you need to assign it coordinates in columns and rows so that we know from which particular column and row line the grid cell will start and on which column and row line it will end. The grid cell coordinates in the illustration in the code above are described as follows:
/*
The cell
starts on column line 4,
ends on column line 5,
starts on row line 3,
ends on row line 4.
*/
.element {
grid-column-start: 4;
grid-column-end: 5;
grid-row-start: 3;
grid-row-end: 4;
}
It is possible to count the coordinates not just from the start, but also from the end of the grid. We can also add a minus sign to the line index that determines where the count starts. The coordinates of the same cell can be described as follows:
/*
The cell
starts on column line 2 counted from the end of the grid,
ends on column line 1 counted from the end of the grid,
starts on row line 3 counted from the end of the grid,
ends on row line 2 counted from the end of the grid.
*/
.element {
grid-column-start: -2;
grid-column-end: -1;
grid-row-start: -3;
grid-row-end: -2;
}
There is also a shorthand syntax for these properties. The grid-column
property combines two properties at once: grid-column-start / grid-column-end
.
Example:
grid-column: 1 / 3;
/* This is the same thing as: */
grid-column-start: 1;
grid-column-end: 3;
Similarly, the grid-row
property is a shorthand property that assigns the following pair of properties: grid-row-start / grid-row-end
.
Example:
grid-row: 1 / -2;
/* This is the same as: */
grid-row-start: 1;
grid-row-end: -2;
If you do not assign a second parameter in the grid-row
or grid-column
property, then the value will remain valid, but only the first parameter will be applied.
Grid items can overlap each other. When this happens, they start to behave as if they were assigned an absolute position. At the same time, they can also be influenced by the z-index
. The greater the z-index
value, the higher the grid item in the stack.
Continue